Apendix 4: Binary Numbers
Our desktop or laptop computers are binary computers. This means that everything is represented within our computers as a number made up of just two digits. To us humans brought up from babyhood counting with a number system based upon 10, a binary number system sounds like an unnecessary complication. However, when it comes to constructing a computer, a binary system is a massive simplification. Binary computers can use a high voltage (actually very low, maybe 3.3 volts) to denote one digit and a zero voltage to denote the other.
There is a general assumption that our number system is based upon 10 because most of us have 10 fingers and our fingers would be a logical start point for counting things. On that basis, a world of cartoon characters would probably have a number system based upon 8 and if (say) dolphins have developed a number system then it might well be based upon 2 like our computers. The chances are high that any alien species in other parts of our galaxy would have a number system based upon their own anatomy. There is no universal law that says 10 is a better system than any other, it just seems the most obvious to us humans.
When we come to write our decimal (base 10) numbers down we find that we only need symbols to represent the digits 0 through 9. This is because the way we represent numbers is a place based system. When we see the number 123 written down we understand that the 1 represents one hundred and this is a greater quantity than the next digit 2 which is also representing a greater quantity (twenty) than the last digit 3. The relative position of each digit tells us what it represents.
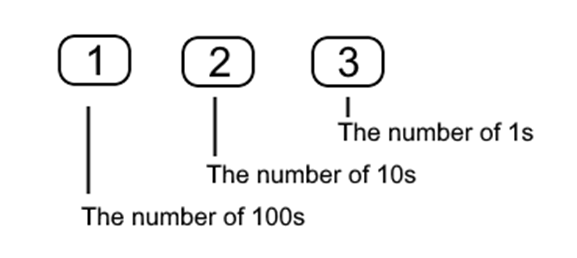
If we wrote down the number 32767, we would know that the 3 represented 3 x 10,000 and the 2 represented 2 x 1,000 and so on to the final 7 which represents 7 x 1. We could also express those position multipliers as powers of 10.
3 | 3 x 10,000 | 3 x 10⁴ |
2 | 2 x 1,000 | 2 x 10³ |
7 | 7 x 100 | 7 x 10² |
6 | 6 x 10 | 6 x 10¹ |
7 | 7 x 1 | 7 x 10⁰ |
(Any number to the power of zero is one by the way.)
A notional cartoon character number system would only need the digits 0 through 7 and a similar position rule could be applied. Numbers base 8 are known as octal.
3 | 3 x 4,096 | 3 x 8⁴ |
2 | 2 x 512 | 2 x 8³ |
7 | 7 x 64 | 7 x 8² |
6 | 6 x 8 | 6 x 8ⁱ |
7 | 7 x 1 | 7 x 8⁰ |
The number 32767 base 8 represents a smaller quantity than 32767 base 10. You might be able to calculate that 32767 base 8 would represent the same value as 13,815 base 10. With a bit more work you might calculate that 32767 base 10 would be represented by the number 77777 base 8.
If the position of a digit in a number tells us how much to multiply that digit by and that this can always be represented as a power of the number base, how would things look for a base 2 system where we only have the digits 0 and 1?
1 | 1 x 128 | 1 x 2⁷ |
0 | 0 x | 0 x 2⁶ |
1 | 1 x 32 | 1 x 2⁵ |
0 | 0 x 16 | 0 x 2⁴ |
1 | 1 x 8 | 1 x 2³ |
1 | 1 x 4 | 1 x 2² |
1 | 1 x 2 | 1 x 2¹ |
1 | 1 x 1 | 1 x 2⁰ |
Clearly, it can work just the same – we just need a few more characters to represent larger numbers. The number 10101111 in binary would be represented as 257 in octal and 175 base 10 (decimal).
How does a binary system work when you need to do arithmetic? Well addition is pretty straightforward. 0 plus 0 is 0. 0 plus 1 is 1 and 1 plus 1 is 0 carry 1. So:
1011001 + 10101 ----------- 1101110
Multiplication is even simpler. Multiply any digit by 0 and the result is 0. Multiply any digit by 1 and it stays the same.
1101 x 1011 ------------ 1101 1101 0000 1101 ======== 10001111
Suppose we had evolved with 8 digits on each hand? If we ignore the challenge this might have given professional cartoonists, we might surmise that humans with that many fingers and thumbs would have developed a counting system with a number base 16. I n fact, base 16 is a very handy number base for programmers and it is known as hexadecimal. We ran into hexadecimal numbers when looking at colour codes earlier in this book. A byte (8 binary digits) is easily represented by two hexadecimal digits and two characters are lot simpler to remember and to type into a program than 8 zeros and ones.
A hexadecimal number system clearly needs digits to represent numbers from zero to fifteen. Being a naturally decimal species we only have digits for zero to nine so programmers have had to call on the services of the letters a to f to fill in for the missing digits. The next table is a bit more challenging as it uses two of the additional digits for decimal values 10 through 15.
E | E x 4096 | E x 16³ | In decimal 14 x 16³ |
7 | 7 x 256 | 7 x 16² | |
C | C x 16 | C x 16¹ | In decimal 12 x 16¹ |
1 | 1 x 1 | 1 x 16⁰ |
e7c1 (upper or lower case is optional) in hexadecimal would be represented as 59,329 in decimal.
Hexadecimal FF (or ff) would be represented as 255 in decimal or 11111111 in binary.
Within a binary computer, all data is represented as a sequence of binary digits. Each binary digit is called a bit and bits are organised as bytes with each byte being constructed from 8 bits. All data is represented by one or more byte. Text typed into a word processing program is translated into numbers using an encoding scheme just as images or MP3 tracks or video clips are represented as a sequence of binary digits. Even the instructions that a microprocessor chip is given to execute (when translated from JavaScript) to manipulate those text, image or media files are simply binary numbers.
Many programming languages have an integer data type, usually in a range of sizes. Integers 8 bits (one byte) long can be used to store numbers in the range -128 to +127 or an unsigned number (no negatives) in the range 0 to 255. A 16 bit integer type might hold integers in the range -32,768 to + 32,767 or unsigned numbers in a range from 0 to 65,535. Signed integers in languages that support the type use one of the available bits to hold the sign and this reduces the maximum (and minimum) value to what can be supported by the remaining binary bits. JavaScript has no direct support for the integer data type except as part of some special array types or when doing bit manipulation.
The last project in this book makes use of the Uint8Array and Uint32Array types which can be used to store unsigned 8 bit integers and unsigned 32 bit integers respectively. Similar data types for signed and 16 bit integers are available.